This tutorial shows how you can simplify reactive Java applications that persist data using the Hibernate ORM with Panache extension in Quarkus.
Business applications preserve valuable business data in persistence stores such as relational databases. The application's presentation layer usually showcases the data for multiple uses, such as inventory, shopping, subscription, and monitoring. Java provides a core feature, the Java Persistence API (JPA), to manage persistent objects using object-relational mapping (ORM) with databases such as PostgreSQL, MySQL, and Microsoft's SQL Server. However, even when using JPA annotations, you must implement the JPA specifications to handle data transactions.
Hibernate ORM with PanacheHibernate ORM with Panache
Hibernate ORM is designed to solve this problem by providing an object-relational mapping framework between Java domain bean classes and schemas in a relational database. The Quarkus framework provides the Hibernate ORM with Panache extension to simplify the persistence layer implementation.
Benefits
Hibernate ORM with Panache offers the following benefits over previous options:
- ID auto-generation
- No need for getters/setters
- Static methods provided for access, such as
listAll
,findById
, andfind
- No need for custom queries for basic operations
Hibernate Reactive
With the rise of the event-driven architecture, Java developers also need to implement these JPA capabilities in a reactive way to improve application performance and scalability on the event-driven architecture. Hibernate Reactive has recently released version 1.0 with a reactive API for Hibernate ORM support, performing non-blocking interactions with relational databases. The Hibernate Reactive API also lets you manage reactive streams using the SmallRye Mutiny programming library.
Note: The code for this tutorial is stored in my GitHub repository.
Add reactive extensions to a Quarkus projectAdd reactive extensions to a Quarkus project
Run the following Maven command in your project directory to add reactive extensions:
$ ./mvnw quarkus:add-extension -Dextensions=resteasy-reactive,resteasy-reactive-jackson,hibernate-reactive-panache,reactive-pg-client
Make sure that the extensions are added to the pom.xml
file in the project directory.
Develop a reactive application with Hibernate Reactive
The Quarkus dev service for PostgreSQL will pull a PostgreSQL container image and run it automatically in your local environment. Be sure to start a container engine before running Quarkus in Dev Mode. Then, to use the live coding feature, run Quarkus Dev Mode through the following Maven command in the project directory:
$ ./mvnw quarkus:dev
The following log messages appear when Quarkus Dev Mode is ready for live coding:
-- Tests paused Press [r] to resume testing, [o] Toggle test output, [h] for more options>
Create a Fruit.java
class file in the src/main/java/{Package path}
directory. Add the following code to create a new entity bean using Hibernate ORM with Panache:
@Entity
@Cacheable
public class Fruit extends PanacheEntity {
public String name;
public Fruit() {}
public Fruit(String name) {
this.name = name;
}
}
Create a new resource class file (e.g., ReactiveGreetingResource
) or update an existing resource class file by adding the following code:
@GET
public Uni<List<Fruit>> listAll() { //<1>
return Fruit.listAll();
}
@GET
@Path("{id}")
public Uni<Fruit> findById(@RestPath Long id) { //<2>
return Fruit.findById(id);
}
@POST
@ReactiveTransactional
public Uni<Fruit> create(Fruit fruit) { //<3>
return Fruit.persist(fruit).replaceWith(fruit);
}
@DELETE
@Path("{id}")
@ReactiveTransactional
public Uni<Void> delete(@RestPath Long id) { //<4>
return Fruit.deleteById(id).replaceWithVoid();
}
Explanations of the annotated lines follow:
- Retrieve all
Fruit
data from the PostgreSQL database using Panache, then return a reactive return stream (Uni
). - Retrieve a particular
Fruit
using Panache, then return a reactive stream (Uni
). - Create a new
Fruit
using a reactive Panache persistence method. - Delete an existing
Fruit
using a reactive Panache persistence method.
Add a few test data items to an import.sql
file in the src/main/resources
directory:
INSERT INTO fruit(id, name) VALUES (nextval('hibernate_sequence'), 'Cherry');
INSERT INTO fruit(id, name) VALUES (nextval('hibernate_sequence'), 'Apple');
INSERT INTO fruit(id, name) VALUES (nextval('hibernate_sequence'), 'Banana');
Go to the terminal where you are running Quarkus in Dev Mode. Press d
to access the Quarkus Dev UI, shown in Figure 1. Take a look at the Hibernate ORM extension box on the right side.
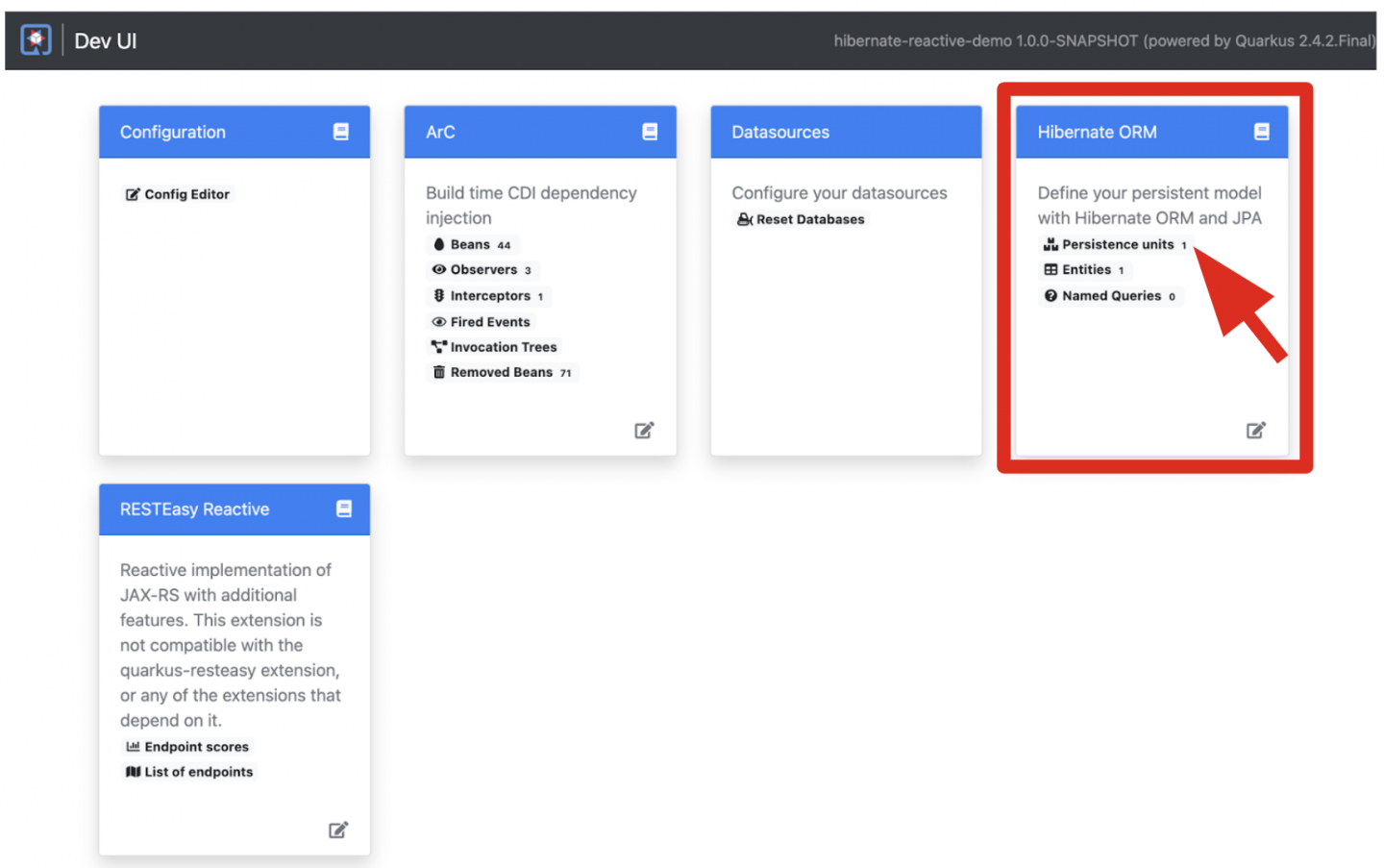
Click Persistence Units in the extension box. The Dev UI displays the SQL statements that can create or drop the data, as shown in Figure 2.
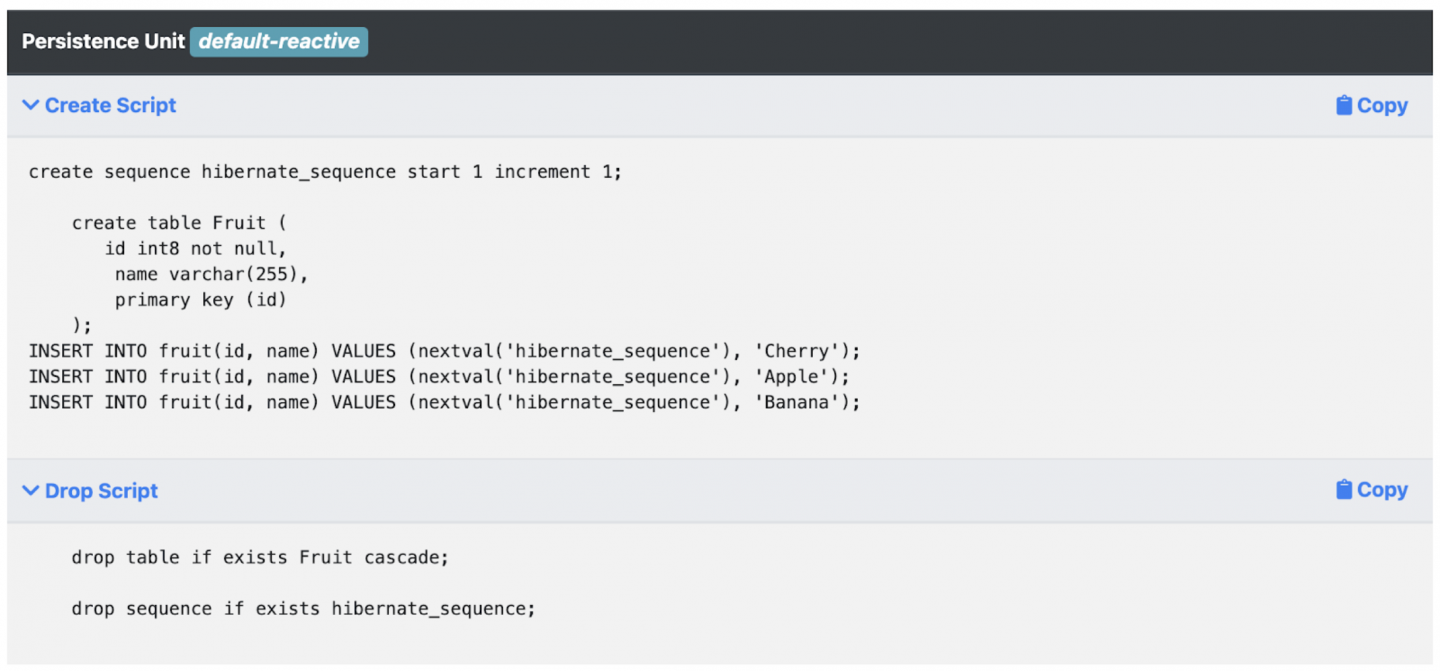
Test the reactive application
Open a new terminal window locally. Invoke the reactive API endpoint using a cURL command or the HTTPie tool. HTTPie could be used as follows:
$ http :8080/fruits
The output should look like this:
HTTP/1.1 200 OK Content-Type: application/json content-length: 75 [ { "id": 1, "name": "Cherry" }, { "id": 2, "name": "Apple" }, { "id": 3, "name": "Banana" } ]
Add new data using the following HTTPie command:
​​​​​$ echo '{"name":"Orange"}' | http :8080/fruits
The output should look like this:
HTTP/1.1 201 Created Content-Type: application/json content-length: 24 { "id": 4, "name": "Orange" }
Now the Orange
appears in the fruits
data:
$ http :8080/fruits HTTP/1.1 200 OK Content-Type: application/json content-length: 100 [ { "id": 1, "name": "Cherry" }, { "id": 2, "name": "Apple" }, { "id": 3, "name": "Banana" }, { "id": 4, "name": "Orange" } ]Where to learn more
Where to learn more
This guide has shown how Quarkus lets you simplify JPA implementation through the Hibernate ORM with Panache extension. Quarkus also provides awesome features to improve developers’ productivity through continuous testing, the Quarkus command-line interface (CLI), the Dev UI, and Dev Services. Find additional resources at the following articles:
Last updated: October 18, 2023