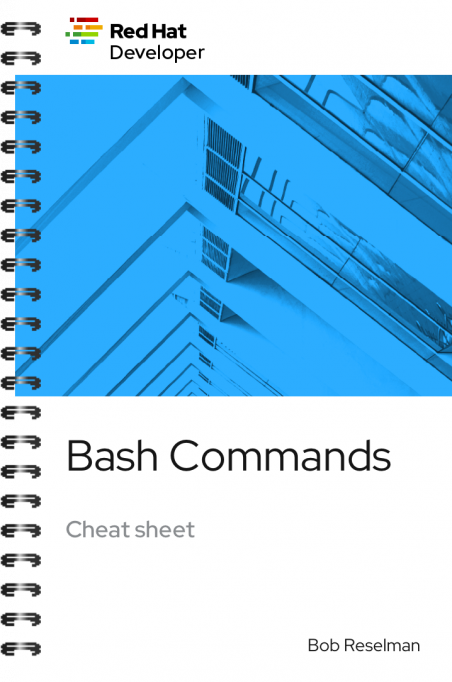
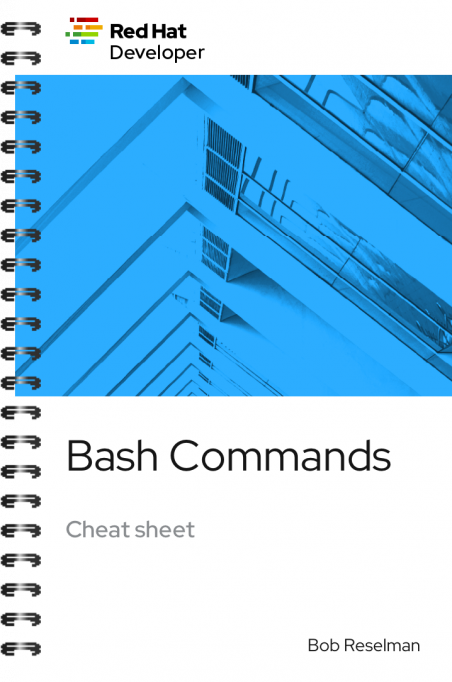
About
Bash is a command language interpreter: a version of the classic Unix shell with many enhancements. Bash is the default shell installed on GNU/Linux distributions and many other Unix-style systems, such as macOS.
Although most developers have a working knowledge of Bash for everyday interactive use, few know the rich features it offers for writing scripts. It supports most of the statements that other languages provide, such as indexed and associative arrays, foreground/background task execution, pipes, redirections, loops, functions, and Boolean expressions.
Download the Bash Commands Cheat Sheet to get started with Bash scripting. You’ll learn how to:
- Create Bash scripts
- Work with variables
- Manipulate strings using parameter expansion
- Create credentials
- Control script flow with conditionals and loops
- Work with collections, arrays, and maps
With Red Hat Developer cheat sheets, you get essential information right at your fingertips so you can work faster and smarter. Easily learn new technologies and coding concepts and quickly find the answers you need.
Excerpt
While loop
A while loop runs continuously until a certain condition is met. The following code uses the less then or equal to symbol -li to run a loop until the counter variable
x reaches the number
5.
x=1;
while [ $x -le 5 ]; do
echo "Hello World"
((x=x+1))
done
Copy, paste and run in your terminal:
Copy and paste the following code to create and run a Bash script that demonstrates a while loop.
cat << 'EOF' > while-loop-01.sh
#!/usr/bin/env bash
x=1;
while [ $x -le 5 ]; do
echo "Hello World"
((x=x+1))
done
EOF
bash while-loop-01.sh
Working with status codes
Reporting success and error in a Bash script is accomplished using status codes. By convention success is reported by exiting with the number 0. Any number greater than
0 indicates an error. Also, there is a convention for error numbers that is explained in the article Bash command line exit codes demystified.
Using the exit keyword
The following demonstrates a Bash code that returns an error code 22 when the script is executed without a parameter.
if [ -z "$1" ]; then
echo "No parameter";
exit 22;
fi
=
Copy, paste, and run in your terminal:
Copy and paste the following code to create and run a Bash script that returns an error code when the script is executed without a parameter.
cat << 'EOF' > status-code-01.sh
#!/usr/bin/env bash
if [ -z "$1" ]; then
echo "No parameter";
exit 22;
fi
EOF
bash status-code-01.sh
echo $?