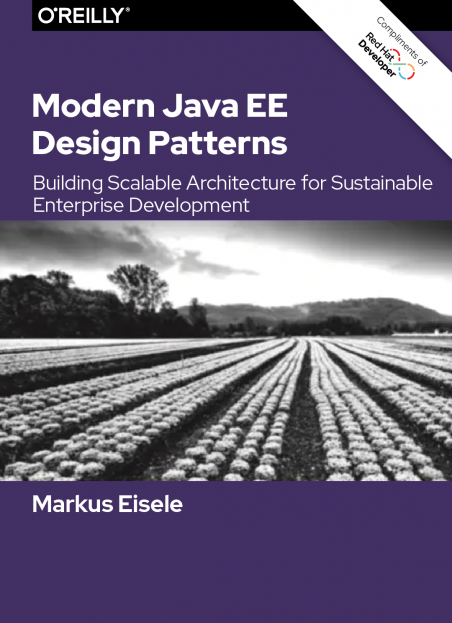
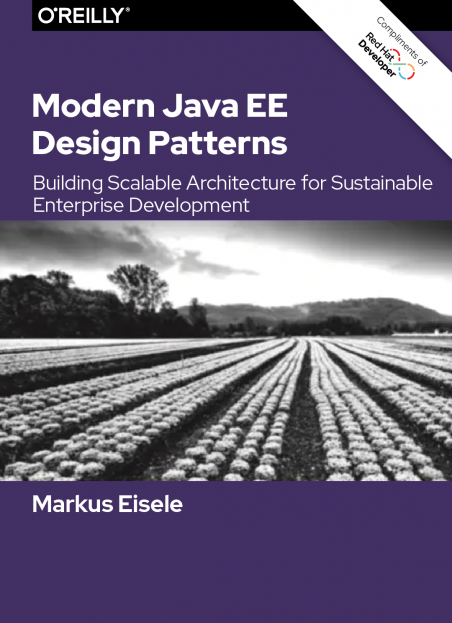
Modern Java EE Design Patterns: Building Scalable Architecture for Sustainable Enterprise Development
Overview
With the ascent of DevOps, microservices, containers, and cloud-based development platforms, the gap between state-of-the-art solutions and the technology that enterprises typically support has greatly increased. But as explained in this O’Reilly report, some enterprises are now looking to bridge that gap by building microservice-based architectures on top of Java EE.
Can it be done? Is it even a good idea? This report thoroughly explores the possibility and provides savvy advice for enterprises that want to move ahead. The issue is complex: Java EE wasn’t built with the distributed application approach in mind, but rather as one monolithic server runtime or cluster hosting many different applications. If you’re part of an enterprise development team investigating the use of microservices with Java EE, there are several items to consider:
- Understand the challenges of starting a greenfield development vs tearing apart an existing brownfield application into services
- Examine your business domain to see if microservices would be a good fit
- Explore best practices for automation, high availability, data separation, and performance
- Align your development teams around business capabilities and responsibilities
- Inspect design patterns such as aggregator, proxy, pipeline, or shared resources to model service interactions
Excerpt
This ebook focuses on how enterprises work and how the situation can be improved by understanding how and when to adopt the latest technologies in such an environment. The main emphasis is on understanding Java EE design patterns, as well as how to work with new development paradigms, such as microservices, DevOps, and cloud-based operations.
This report also introduces different angles to the discussion surrounding the use of microservices with well-known technologies, and shows how to migrate existing monoliths into more fine-grained and service-oriented systems by respecting the enterprise environment. As you’ll come to find out, Java EE is only a very small — yet crucial — component of today’s enterprise platform stacks.