The first article in this series introduced the challenges of modernizing a monolithic, legacy Java application, and we deployed our example Java EE 8 application on Red Hat JBoss Enterprise Application Platform (JBoss EAP). This article guides you through the first of three steps to carry out the modernization to a cloud-ready Java application.
The changes in this article do not yet involve containers, microservices, or other elements of application modernization. We'll cover those steps in Part 3 and Part 4. Here, we'll set the stage by upgrading our Java environment as follows:
- From Java 8 to Java 11
- From JBoss EAP 7.2 to JBoss EAP 7.3
- From Java to Jakarta EE
The weather-app-eap-cloud-ready
subproject, which you retrieved from my GitHub repository in the previous article, contains the configuration file that implements these changes. The advantages of this upgrade will be particularly apparent when you arrive at the final article in the series.
Read the whole series:
-
Part 1: An incremental approach using Jakarta EE and MicroProfile
-
Part 2: Upgrade the legacy Java application to Jakarta EE
-
Part 3: Integrate MicroProfile services
-
Part 4: Optimize the runtime environment
Upgrade from Java 8 to Java 11
One of the benefits of using standards is that you do not need to rewrite your application in order to evolve it. Moving from Java 8 to Java 11 is important because Java 11 contains improvements to make the Java workload more efficient in a container environment. To use Java 11 in our example application, we only need to change the maven.compiler.source
and maven.compiler.target
properties in our Maven pom.xml
file.
The version of the application from Part 1 contained the following:
<properties>
...
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
...
</properties>
The new file is updated as follows:
<properties>
...
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
...
</properties>
Upgrade to JBoss EAP 7.3 and Jakarta EE
Next, we need to update our application to use JBoss EAP 7.3, which has a long list of fixes and improvements. In this step, we will also upgrade the application to use Jakarta EE.
Note: Why use Jakarta EE? Oracle donated Java EE to the Eclipse Foundation with a "recommendation" for a new name and branding. The transition was quite long, but now the organization is ready to guarantee the evolution of the specifications to make them more attractive for developers and ready to be used in a cloud environment.
To use Jakarta EE in our application, we need to update our application's server version from JBoss EAP 7.2 to JBoss EAP 7.3. We'll then declare the right dependencies in pom.xml
, moving from the javax
to the jakarta
namespace:
...
<properties>
...
<version.server.bom>7.3.5.GA</version.server.bom>
...
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.jboss.bom</groupId>
<artifactId>jboss-eap-jakartaee8</artifactId>
<version>${version.server.bom}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>jakarta.persistence</groupId>
<artifactId>jakarta.persistence-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>jakarta.enterprise</groupId>
<artifactId>jakarta.enterprise.cdi-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.jboss.spec.javax.ws.rs</groupId>
<artifactId>jboss-jaxrs-api_2.1_spec</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.eclipse.microprofile.health</groupId>
<artifactId>microprofile-health-api</artifactId>
<version>1.0.0.redhat-83</version>
</dependency>
</dependencies>
....
Configure the JDBC driver for PostgreSQL
The last thing to do is to configure the driver the Java Database Connectivity API (JDBC) needs to interact with our PostgreSQL database. JBoss EAP 7.3 removed the data sources provided in the previous release of Red Hat OpenShift's image, so we need to add a bit of our own configuration. I created a custom directory with the name .s2i
(starting with a dot). The directory contains just a file named environment
with the following key/value definition:
CUSTOM_INSTALL_DIRECTORIES=extensions
This file will be used in the Source-to-Image (S2I) process to identify the project’s path, so it can find the resources needed to make the JDBC driver and the configuration of the data source available to the application server.
The project structure is based on the following files in the extensions
directory:
drivers.env
: The file that contains the information about the JDBC drivers.install.sh
: The file that contains the instructions that the S2I process will use to create the JDBC driver as a JBoss EAP module, along with the data sources' configurations.modules
: The directory that contains the module structure that will be put into the JBoss EAP image.
That’s all that is required to make the upgrades: We don’t need to touch the Java code. You might be thinking that this clean approach was possible because the application is a simple one, but in more complex applications the custom sections are built using third-party libraries. Upgrading the average application and its server subsystem according to the Java EE or Jakarta EE specifications can easily be done without touching the code. Once you've done the upgrade, you are able to use the new functionalities provided by the application server.
Deploy the new Jakarta EE release
Okay, now it’s time to deploy the new release to verify that everything continues to work well. The steps are as follows:
-
Import the image for JBoss EAP 7.3 with OpenJDK 11:
$ oc import-image jboss-eap-7/eap73-openjdk11-openshift-rhel8 --from=registry.redhat.io/jboss-eap-7/eap73-openjdk11-openshift-rhel8 --confirm
-
Create the build related to the cloud-ready weather app that will be deployed on JBoss EAP 7.3:
$ oc new-build eap73-openjdk11-openshift-rhel8 --binary=true --name=weather-app-eap-cloud-ready
-
Move to the
weather-app-eap-cloud-ready
directory and start the new build:$ cd ../weather-app-eap-cloud-ready $ oc start-build weather-app-eap-cloud-ready --from-dir=. --wait
-
Create the new release of the application:
$ oc new-app weather-app-eap-cloud-ready -e DB_SERVICE_PREFIX_MAPPING=weatherds-postgresql=DB \ -e DB_JNDI=java:jboss/datasources/WeatherDS \ -e DB_DATABASE=weather \ -e DB_USERNAME=mauro \ -e DB_PASSWORD=secret \ -e DB_DRIVER=postgresql \ -e DB_NONXA=true \ -e DB_URL='jdbc:postgresql://$(WEATHER_POSTGRESQL_SERVICE_HOST):$(WEATHER_POSTGRESQL_SERVICE_PORT)/weather'
-
Expose the route in order to test the application:
$ oc expose svc weather-app-eap-cloud-ready
Verify the application works on OpenShift
Go to the OpenShift console, as shown in the previous article in this series. Select the weather-app-eap-cloud-ready icon, click the route for the application, and verify that the new version of the application works. Figure 1 shows statistics for the original, traditional application.

Figure 2 shows statistics for the new version we've just created.
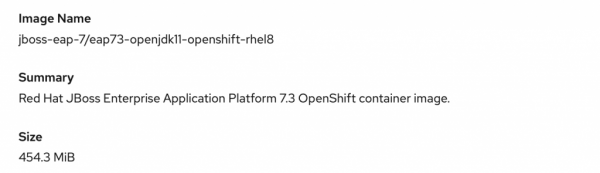
Conclusion to Part 2
You have updated your application without changing the code. With the updates in this article, you've achieved the following:
- The example application has new features provided by Java 11.
- You can also access newer functionalities provided by JBoss EAP 7.3.
- You've laid the groundwork for upgrading to future Jakarta EE specification updates.
A small side-effect of the upgrades is that the image of JBoss EAP 7.3 application is larger than the previous version. JBoss EAP 7.3 has more features, so a size increase can be expected. From an application perspective, it's a waste, however, because we're using the same functionalities as before. In the final article of this series, I will show you how to drastically reduce the application's size and memory footprint. Before we do that, we'll add a bunch of tools that we need for a distributed, containerized application.
Next up: Part 3: Integrate MicroProfile services.
Last updated: January 12, 2024