The goal of the Drogue IoT project is to make it easy to connect devices and cloud-based applications with each other. In this article, we'll show how to implement firmware based on Drogue Device that can communicate with Quarkus applications in the cloud using the low-power LoRaWAN protocol.
Drogue IoTDrogue IoT
A lot of open source technology already exists in the realm of messaging and IoT (Internet of Things), so it makes sense to try to use as many existing tools as possible. However, technologies change over time, and not everything that exists now is fit for the world of tomorrow. C and C++ still have issues with memory safety, and cloud native, serverless, and pods are concepts that might need a different approach when designing cloud-side applications. So, Drogue IoT is here to help.
Drogue Device is a firmware framework written in Rust with an actor-based programming model. Drogue Cloud is a thin layer of services that creates an IoT-friendly API for existing technologies like Knative and Apache Kafka and provides a cloud-friendly API using CloudEvents on the other side. The idea is not to provide separated components but to give you an overall solution that's ready to run: IoT as a service. The Drogue IoT architecture is shown in Figure 1.
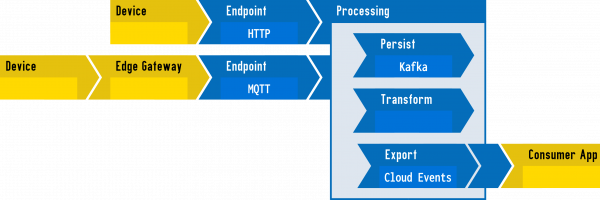
LoRaWAN network coverage
LoRaWAN is a low-power wireless network that enables you to run a device on batteries for months, sending telemetry data to the cloud every now and then. To accomplish that, you need LoRaWAN network coverage, and The Things Network (TTN) provides exactly this. You can extend the TTN network by running your own gateway, should your local area lack coverage. TTN provides a public service that allows you to exchange data between devices and applications.
Drogue DeviceDrogue Device
Exchanging data with Drogue Device is easy. The following snippet focuses on the actual code exchanging data:
let mut tx = String::<heapless::consts::U32>::new();
let led = match self.config.user_led.state().unwrap_or_default() {
true => "on",
false => "off",
};
write!(&mut tx, "ping:{},led:{}", self.counter, led).ok();
let tx = tx.into_bytes();
let mut rx = [0; 64];
let result = cfg
.lora
.request(LoraCommand::SendRecv(&tx, &mut rx))
.unwrap()
.await;
Notice the await
keyword? Yes, that is indeed asynchronous Rust running on an embedded STM32 Cortex-M0 device. Thanks to the embassy framework and the drivers in Drogue Device, asynchronous programming becomes pretty simple. And thanks to Rust, your code is less likely to cause any undefined behavior, like corrupted memory.
Quarkus
On the cloud side, we want to have a simple "reconcile loop." The device reports its current state, and we derive the desired state from that. This might result in a command that we want to send back to the device. Again, focusing on the code that is important:
@Incoming("event-stream")
@Outgoing("device-commands")
@Broadcast
public DeviceCommand process(DeviceEvent event) {
var parts = event.getPayload().split(",");
// then we check if the payload is, what we expect...
if (parts.length != 2 || !parts[0].startsWith("ping:") || !parts[1].startsWith("led:")) {
// .. if not, return with no command
return null;
}
// check if the configured response is about the LED, and if it matches ...
if (!this.response.startsWith("led:") || parts[1].equals(this.response)) {
// ... it is not, or it matches, so we return with no command
return null;
}
var command = new DeviceCommand();
command.setDeviceId(event.getDeviceId());
command.setPayload(this.response.getBytes(StandardCharsets.UTF_8));
return command;
}
Pretty simple, isn't it? Of course, you could make it more complicated, but we leave that up to you.
The Quarkus application consumes CloudEvents, which provide a standardized representation of events for different messaging technologies like Kafka, HTTP, and MQTT.
Drogue CloudDrogue Cloud
So far, this is pretty straightforward, focusing on the actual use case. However, we are missing a big chunk in the middle: How do we connect Quarkus with the actual device? Sure, we could recreate all of that ourselvesâimplementing the TTN API, registering devices, processing events. Alternatively, we could simply use Drogue Cloud and let it do the plumbing for us.
Creating a new application and device is easy using the drg
command-line tool:
$ drg create application my-app
$ drg create device --app my-app my-device
The device registry in Drogue Cloud not only stores device information but can also reconcile with other services. Adding the following information will make it sync with TTN:
$ drg create application my-app --spec '{
"ttn": {
"api": {
"apiKey": "...",
"owner": "my-ttn-username",
"region": "eu1"
}
}
}'
$ drg create --app my-app device my-device --spec '{
"ttn": {
"app_eui": "0123456789ABCDEF",
"dev_eui": "ABCDEF0123456789",
"app_key": "0123456789ABCDEF...",
"frequency_plan_id": "...",
"lorawan_phy_version": "PHY_V1_0",
"lorawan_version": "MAC_V1_0"
}
}'
This creates a new TTN application, registers the device, sets up a webhook, creates the gateway configuration in Drogue Cloud, and ensures that credentials are present and synchronized.
Learn more in the LoRaWAN end-to-end workshopLearn more in the LoRaWAN end-to-end workshop
Did that seem a bit fast? Yes, indeed! This is a lot of information for a single article, so we wanted to focus on the most important parts. We put together everything you need to know in a workshop so you can read through it in more detail and get more background, too. By the end of this workshop, you should end up with a web front-end to control your device, as shown in Figure 2.
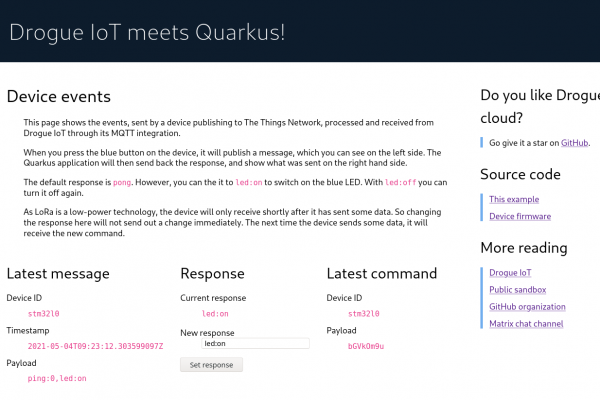
Most importantly, you'll have a solid foundation for creating your own applications on top of Drogue IoT.
Last updated: June 28, 2023