Page
Add metrics to your applications
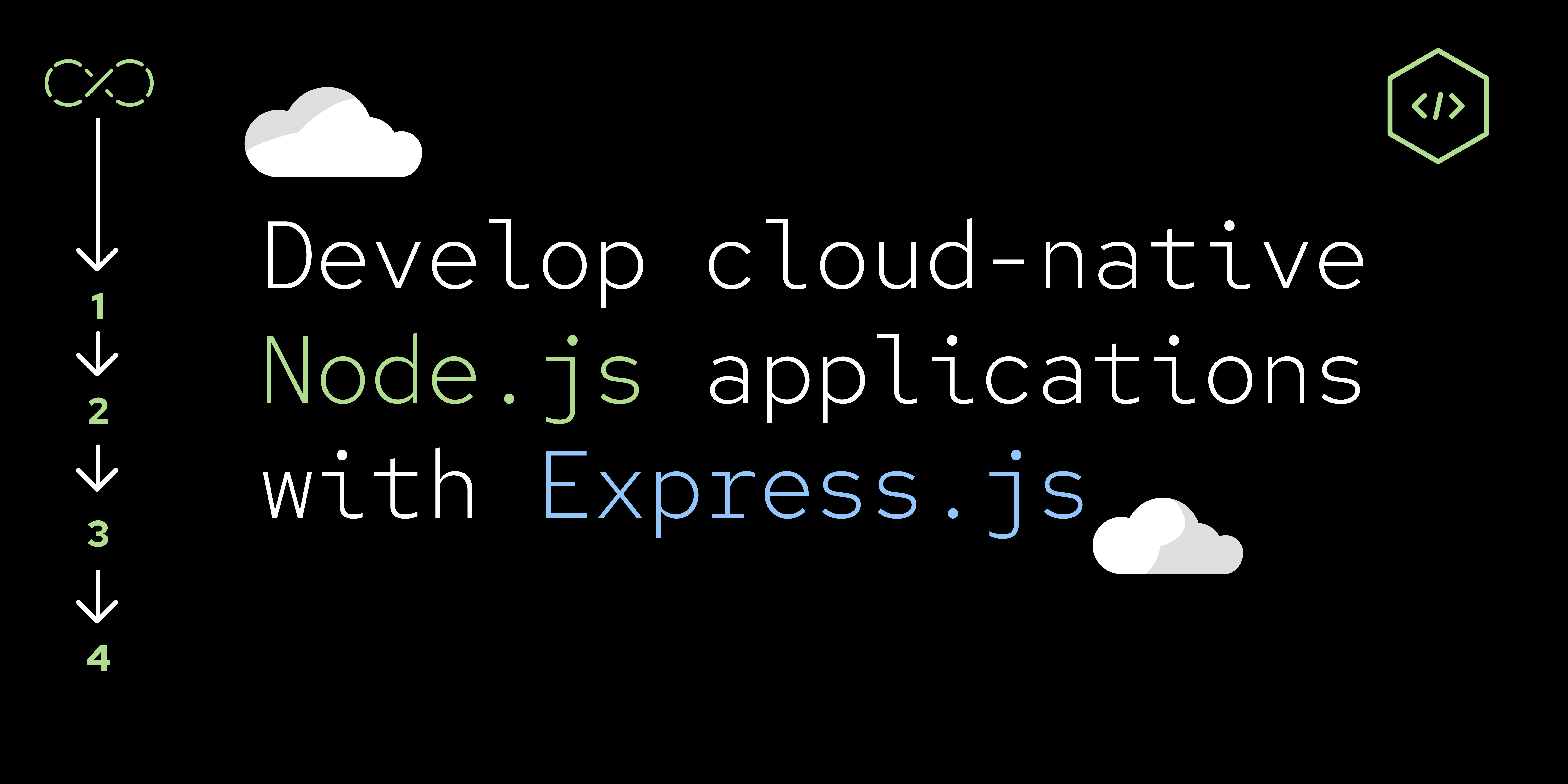
For any application deployed to a cloud, it is important that the application is "observable:" that you have sufficient information about an application and its dependencies such that it is possible to discover, understand, and diagnose the state of the application. One important aspect of application observability is metrics-based monitoring data for the application.
One of the Cloud Native Computing Foundation (CNCF)-recommended metrics systems is Prometheus, which works by collecting metrics data by making requests of a URL endpoint provided by the application. Prometheus is widely supported inside Kubernetes, meaning that Prometheus also collects data from Kubernetes itself, and application data provided to Prometheus can also be used to automatically scale your application.
The prom-client
package provides a library that auto-instruments your application to collect metrics. It is then possible to expose the metrics on an endpoint for consumption by Prometheus.
In order to get full benefit from taking this lesson, you need:
- The application from the previous lessons.
- An environment where you can install and run a Node.js application.
In this lesson, you will:
- Install the
prom-client
module. - Set up an endpoint to expose metrics.
Add metrics to your application
The first step to setting up metrics-based monitoring data for your application is adding the prom-client module to the project.
Add the prom-client module to the project.
npm install prom-client
Now, require prom-client in server.js and choose to configure default metrics.
// Prometheus client setup
const Prometheus = require('prom-client');
Prometheus.collectDefaultMetrics();
It is recommended to add these lines below the Pino logger import.
Next, register a /metrics
route to serve the metrics data on:
app.get('/metrics', async (req, res, next) => {
try {
res.set('Content-Type', Prometheus.register.contentType);
const metrics = await Prometheus.register.metrics();
res.end(metrics);
} catch {
res.end('');
}
});
Register the app.get('/metrics')...
route after your /live
route handler. This adds a /metrics
endpoint to your application and automatically starts collecting data from your application and exposes it in a format that Prometheus understands.
Now, restart your application.
npm start
And finally, visit the metrics endpoint: http://localhost:8080/metrics
You can stop your server by entering Ctrl + C in your terminal window.
For information on how to configure the prom-client
library, see the prom-client documentation.
And you’re done! You have successfully used prom-client
to add metrics-based monitoring to your application.
In the next lesson, you will deploy your application on the Developer Sandbox.