As developers, we don't always consider test migration when we think about adopting a new framework. Tests are important, however, because they ensure that our code meets its requirements and works as desired, especially when we add new features and functionality.
Test migration is an essential part of migrating to a new application development framework. This article is for developers who are migrating a Spring Boot application to Quarkus. I will use three sample tests to demonstrate a test migration from Spring Boot to Quarkus. While Quarkus is compatible with Spring Boot Web, not all of Spring Boot's test functionalities map to Quarkus. I will introduce you to other test dependencies and Quarkus capabilities that you can use in these cases.
What is Quarkus? Quarkus is a full-stack, Kubernetes-native Java framework made for Java virtual machines and native compilation. Spring Boot optimizes Java for containers, making it an effective platform for serverless, cloud, and Kubernetes environments.
Getting started with a test migration
The Quarkus community site contains detailed documentation about the different ways to write tests for Quarkus applications. I used this documentation as a basis for migrating a Spring Boot sample application to Quarkus. I also used test examples from the Quarkus GitHub repository.
The sample application
Our Spring Boot sample application allows employees to complete surveys where they rate the skills of other employees assigned to the same project. Our task is to migrate the application tests from Spring Boot to Quarkus. To get started, check out the source code for the Spring Boot application and the Quarkus application.
Note: For the examples, I assume that you have already started the process of migrating a Spring Boot app to Quarkus. I won't offer much detail about either framework. The focus of this article is test migration.
Test dependencies
For this migration, we will use the JUnit 5 and REST Assured test dependencies, as shown in the Maven pom.xml
below:
<dependency> <groupId>io.quarkus</groupId> <artifactId>quarkus-junit5</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>io.rest-assured</groupId> <artifactId>rest-assured</artifactId> <scope>test</scope> </dependency>
Test folder structure
Figure 1 shows the current structure of the tests:
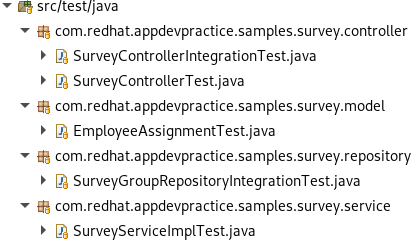
Example 1: SurveyControllerIntegrationTest.java
For each of our Spring Boot tests, we'll need to change the annotations to be compatible with Quarkus. Notice the annotations in our first example, SurveyControllerIntegrationTest.java
:
@SpringBootTest @AutoConfigureMockMvc @ActiveProfiles(“test”) public class SurveyControllerIntegrationTest { @Autowired private MockMvc mockMvc; @Autowired private ObjectMapper mapper; @Autowired private SurveyGroupRepository surveyGroupRepository; …
Quarkus does not need the @SpringBootTest
annotation, and it doesn't support @AutoConfiguremockMvc
. Instead, tests in Quarkus are annotated with @QuarkusTest
. Tests that interact with the database are annotated with @Transactional
. In the sample below, we remove the @Autowired MockMvc
MVC annotation. Also note that instead of using Spring Boot's @Autowired
on the repository, Quarkus uses @Inject
:
@Transactional @QuarkusTest public class SurveyControllerIntegrationTest { @Autowired private ObjectMapper mapper; @Inject private SurveyGroupRepository surveyGroupRepository; …
Migrating from MockMvc to REST Assured
As I mentioned, Quarkus does not support MockMvc
. While it does have other mocking capabilities, it's best to use REST Assured to test the controller in Quarkus. REST Assured is a Java library that allows you to test APIs using a domain-specific language (DSL).
In this first example, you can see how Spring Boot uses MockMvc
to test a POST
survey-group request:
@Test public void shouldPersistASurveyGroup() throws Exception { MvcResult result = mockMvc.perform(post(“/surveygroups”) .contentType(MediaType.APPLICATION_JSON) .content(mapper.writeValueAsString(ResourceHelper.getDefaultSurveyGroupResource()))) .andExpect(status().isCreated()) .andReturn(); String locationHeader = result.getResponse().getHeader(“location”); List<SurveyGroup> surveyGroups = surveyGroupRepository.findAll(); SurveyGroup surveyGroup = surveyGroups.get(0); assertTrue(surveyGroups.size() == 1); assertTrue(locationHeader.contains(surveyGroup.getGuid())); }
Here's how you would use REST Assured in Quarkus to write a similar test:
@Test public void shouldPersistASurveyGroup() throws Exception { String location = RestAssured.given().accept(ContentType.JSON).request() .contentType(ContentType.JSON) .body(ResourceHelper.getDefaultSurveyGroupResource()) .when().post(“/surveygroups”).then() .statusCode(201).extract().header(“Location”); String guid = location.toString().replace(“http://localhost:8081/surveygroups/”, “”); assertTrue(repository.findByGuid(guid).isPersistent()); }
As a result, SurveyControllerTest.java
is not necessary when using Quarkus, because its testing can be covered with Rest Assured.
Example 2: SurveyGroupRepositoryIntegrationTest.java
We will need to change the annotations for this Spring Boot test, as well:
@DataJpaTest @ActiveProfiles(“test”) public class SurveyGroupRepositoryIntegrationTest { @Autowired private SurveyGroupRepository surveyGroupRepository; …
Once again, we use the @Inject
annotation instead of @Autowired
:
@Transactional @QuarkusTest public class SurveyGroupRepositoryIntegrationTest { @Inject private SurveyGroupRepository surveyGroupRepository; …
Migrating repository tests to Quarkus
Here is one of the Spring Boot repository tests for this sample:
@Test public void shouldPersistSurveyGroup() { SurveyGroup surveyGroup = new SurveyGroup(); surveyGroup.setGuid(“guid123”); surveyGroup = this.surveyGroupRepository.saveAndFlush(surveyGroup); assertNotNull(surveyGroup.getId()); assertTrue(surveyGroup.getGuid().equals(“guid123”)); }
Notice that the Spring Boot application uses a Java Persistence API (JPA) repository. To optimize this test for Quarkus, we'll change the repository to Panache, a Hibernate ORM (object-relational mapper) that implements JPA. We also have to change a few of the methods in the Spring Boot test. For example, we now have to use surveyGroupRepository.persistAndFlush()
rather than surveyGroupRepository.saveAndFlush()
. Other than these small changes, the majority of the repository tests remain the same:
@Test public void shouldPersistSurveyGroup() { SurveyGroup surveyGroup = new SurveyGroup(); surveyGroup.setGuid(“guid1234”); this.surveyGroupRepository.persistAndFlush(surveyGroup); SurveyGroup sg = surveyGroupRepository.findByGuid(“guid1234”); assertTrue(sg.getGuid().equals(“guid1234”)); }
Example 3: SurveyServiceImpl.java
In this example, we can see that the Spring Boot test uses Mockito, and it also injects mocks:
@ExtendWith(MockitoExtension.class) public class SurveyServiceImplTest { @Mock private SurveyGroupRepository repository; @InjectMocks private SurveyServiceImpl surveyService; …
Quarkus does not support MockitoExtension
, and while Quarkus does have mocking capabilities, we can test the SurveyService
without mocks. To migrate this test to Quarkus, we inject the repository and service, as well as a test resource:
@QuarkusTest @Transactional @QuarkusTestResource(H2DatabaseTestResource.class) public class SurveyServiceImplTest { @Inject private SurveyGroupRepository repository; @Inject private SurveyServiceImpl surveyService; …
No more mocks
Notice that this Spring Boot test uses mocking to see if a surveyGroup
has been created:
@Test public void shouldCreateSurveyGroup() { SurveyGroup surveyGroup = new SurveyGroup(); when(this.repository.saveAndFlush(surveyGroup)).thenReturn(surveyGroup); this.surveyService.createSurveyGroup(surveyGroup); verify(this.repository).saveAndFlush(surveyGroup); }
For our migration, we removed mocking and injected the repository and service instead. So, we can simply create a new instance of a surveyGroup
and call the service methods directly onto it, to test its functionality:
@Test public void shouldCreateSurveyGroup() { SurveyGroup surveyGroup = new SurveyGroup(); this.surveyService.createSurveyGroup(surveyGroup); assertTrue(repository.listAll() != null); }
Conclusion
I hope the three test migration examples in this article have provided a helpful overview for migrating Spring Boot tests to Quarkus. I did not cover all of the tests in the provided sample application, so feel free to reference the Spring Boot GitHub repository to see more test migration examples. I also hope that you will explore the Quarkus test documentation links that I provided throughout the article, as well as the Quarkus test GitHub repository for more examples.
Additional resources
- Quarkus for Spring developers is a good resource for Spring developers considering Quarkus.
- Quarkus for Spring Boot developers is a self-paced tutorial for Spring and Spring Boot developers migrating to Quarkus.
- DevNation Tech Talk: Kubernetes-native Spring apps on Quarkus is a live-coding demonstration of a Quarkus application using popular Spring features.
- For more about migrating Spring Boot apps to Quarkus, see Migrating a Spring Boot microservices application to Quarkus.