In this article, we'll look at using OpenAPI with .NET Core. OpenAPI is a specification for describing RESTful APIs. First, I'll show you how to use OpenAPI to describe the APIs provided by an ASP.NET Core service. Then, we'll use the API description to generate a strongly-typed client to use the web service with C#.
Writing OpenAPI descriptionsWriting OpenAPI descriptions
Developers use the OpenAPI specification to describe RESTful APIs. We can then use OpenAPI descriptions to generate a strongly-typed client library that is capable of accessing the APIs.
Note: Swagger is sometimes used synonymously with OpenAPI. It refers to a widely used toolset for working with the OpenAPI specification.
Build the web service
In this section, we'll use the open source Swashbuckle.AspNetCore package to provide an OpenAPI description of an ASP.NET Core application.
We start by creating a webapi
template application:
$ dotnet new webapi -o WebApi1 $ cd WebApi1
The webapi
template includes a REST API to get a weather forecast. The API is implemented in the WeatherForecastController.cs
file.
Next, we add the Swashbuckle.AspNetCore
package:
$ dotnet add package Swashbuckle.AspNetCore
Now, we make a few edits to the Startup.cs
file:
public void ConfigureServices(IServiceCollection services) { services.AddControllers(); + + services.AddSwaggerGen(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { .... app.UseHttpsRedirection(); + app.UseSwagger(); + + app.UseSwaggerUI(c => + { + c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1"); + }); + app.UseRouting(); app.UseAuthorization();
In the ConfigureServices
method, we call AddSwaggerGen
. Calling AddSwaggerGen
makes the API description available. The API can then be consumed through ASP.NET Core's dependency injection (DI) system. UseSwagger
uses these descriptions to create an HTTP endpoint at /swagger/v1/swagger.json
. The UseSwaggerUI
then provides a user interface (UI) at /swagger
that allows users to easily consume the exposed API from a browser.
Note: The methods called in Startup.cs
accept a delegate for configuration. For useful options, see the ASP.NET Core documentation, Get started with Swashbuckle and ASP.NET Core.
Run the app
You can run the application and browse to the Swagger UI, which is shown in Figure 1.
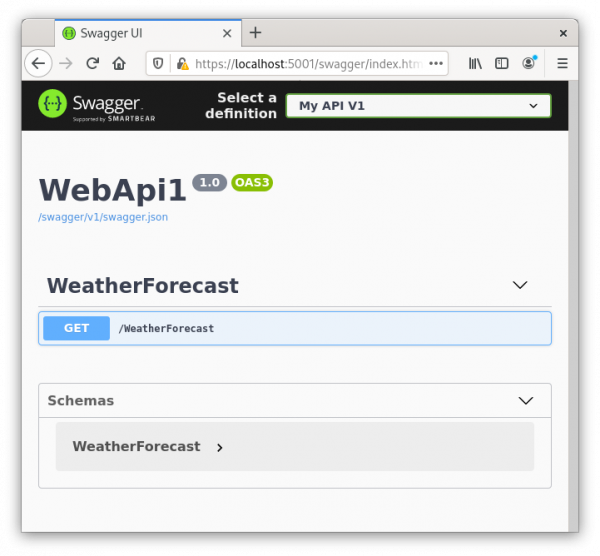
The Swashbuckle.AspNetCore
packages picked up the ASP.NET endpoints. The exposed UI makes it easy to invoke the REST endpoints.
Consuming OpenAPI descriptions
In this section, we'll look at consuming a RESTful API that has an OpenAPI description. To consume the API, we'll use the open source package, NSwag.ApiDescription.Client.
First, we create a new console
project, and download the OpenAPI description from our ASP.NET application:
$ dotnet new console -o console $ cd console $ mkdir openapi $ wget --no-check-certificate https://localhost:5001/swagger/v1/swagger.json -O openapi/weather.json
Now, we'll make a few edits to the project file. These edits will be used to generate a strongly-typed client when the .NET project is built:
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <OutputType>Exe</OutputType> <TargetFramework>netcoreapp3.1</TargetFramework> </PropertyGroup> + <ItemGroup> + <PackageReference Include="Newtonsoft.Json" Version="12.0.2" /> + <PackageReference Include="NSwag.ApiDescription.Client" Version="13.0.5" /> + </ItemGroup> + <ItemGroup> + <OpenApiReference Include="openapi/weather.json" Namespace="WeatherService"> + <ClassName>WeatherClient</ClassName> + <OutputPath>WeatherClient.cs</OutputPath> + </OpenApiReference> + </ItemGroup> </Project>
We've added references to the NSwag.ApiDescription.Client
and Newtonsoft.Json
packages. An OpenApiReference
element refers to the API description that we downloaded earlier. It adds attributes that are required to generate the code, such as the class name, namespace, and filename.
Build the client
Now, we'll invoke the build
command. Invoking the command generates a WeatherClient.cs
file, which lives under the obj
directory:
$ dotnet build
We can now edit the Program.cs
file and use the strongly-typed WeatherClient
class that we've just generated:
static async Task Main(string[] args) { // Configure HttpClientHandler to ignore certificate validation errors. using var httpClientHandler = new HttpClientHandler(); httpClientHandler.ServerCertificateCustomValidationCallback = (message, cert, chain, errors) => { return true; }; // Create WeatherClient. using var httpClient = new HttpClient(httpClientHandler); var weatherClient = new WeatherService.WeatherClient("http://localhost:5000", httpClient); // Call WeatherForecast API. var forecast = await weatherClient.WeatherForecastAsync(); foreach (var item in forecast) { Console.WriteLine($"{item.Date} - {item.Summary}"); } }
Run the app
Finally, we run the application:
$ dotnet run 7/1/2020 1:18:18 PM +02:00 - Mild 7/2/2020 1:18:18 PM +02:00 - Bracing 7/3/2020 1:18:18 PM +02:00 - Freezing 7/4/2020 1:18:18 PM +02:00 - Balmy 7/5/2020 1:18:18 PM +02:00 - Bracing
As you can see, the weather report is mixed.
ConclusionConclusion
In this article, you learned about the OpenAPI specification, which is sometimes used synonymously with Swagger. Developers use the OpenAPI spec to describe RESTful APIs in preparation for being consumed by a client. I showed you how to use the Swashbuckle.AspNetCore package to provide an OpenAPI description of an API implemented using ASP.NET Core. Then, we used the NSwag.ApiDescription.Client package to generate a strongly-typed client capable of consuming the API.
Last updated: February 5, 2024