Page
Use the Developer Sandbox to create your back-end Quote Of The Day web service
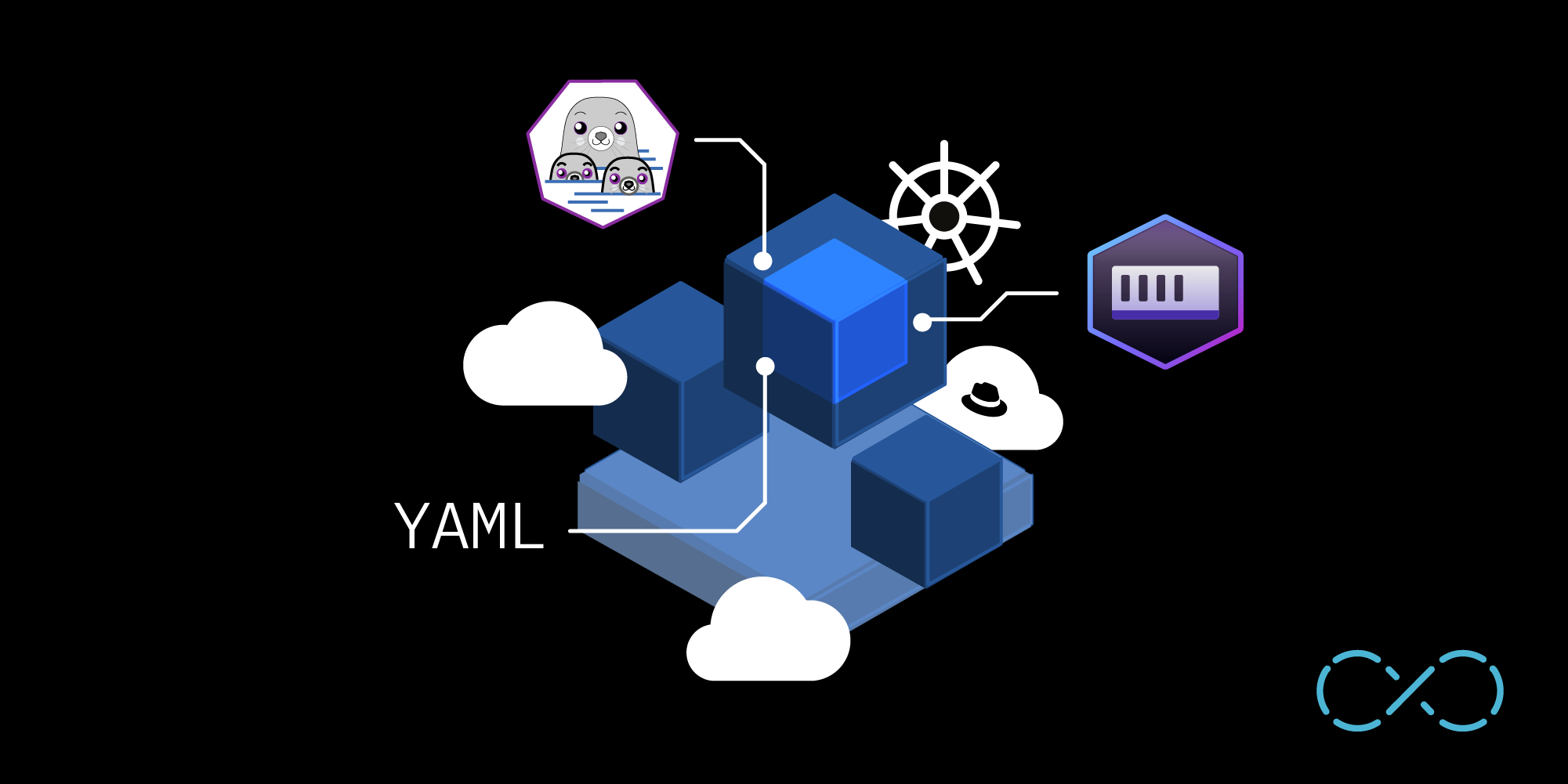
Your first step in the path to injecting variable values at runtime is to create a back-end web service.
What you need
- A Developer Sandbox account
- The
oc
command line interface - A web browser to access your sandbox dashboard
What you will do
- Create a back-end service
- Build a route to the service
- Create a custom ConfigMap
Log into the Developer Sandbox at the command line
If you’re unsure how to log into the Developer Sandbox, you can find instructions here.
Create a back-end Quote Of The Day web service
The backend Quote Of The Day web service, qotd, is a RESTful service. You can find the source code at the qotd GitHub repo. The service has the following endpoints:
Endpoint |
Returns |
\ |
Name of service, i.e. “qotd” |
\quotes |
JSON array of quotes |
\quotes\{quoteId} |
JSON document of a single quote with specific ID |
\quotes\random |
JSON document of a single, random quote |
\writtenin |
String “Go” |
\version |
Version number of service |
- To create this backend service using s2i, run the following command at the command line:
oc new-app https://github.com/redhat-developer-demos/qotd --labels=tier=backend,language=golang,qotd=backend,sandbox=environment-variables
- Create a secure route to the web service. At the command line, run the following command to create a secure route (an external URL) to the new Quote of the Day web service:
oc create route edge --service=qotd --port=10000
- Get the URL to your back-end web service. To get the URL, run the following command at the command line:
oc get route qotd -o jsonpath='{"https://"}{.spec.host}'
Info alert:Save the URL you create. You will need it later.
- Here is an example:
PS C:\> oc get route qotd -o jsonpath='{"https://"}{.spec.host}' https://qotd-rhn-engineering-dsch-dev.apps.sandbox-m3.1530.p1.openshiftapps.com
- To that URL, you will need to append the endpoint at which we need to get a random quote, which is \quotes\random. Using the above example, it would look like this:
https://qotd-rhn-engineering-dsch-dev.apps.sandbox-m3.1530.p1.openshiftapps.com\quotes\random
- Here's how this next part works. The technique you're about to use, involves changing the source code at runtime, just prior to starting the application. In this case, the application is running Nginx to serve up the web pages. Here’s a snippet of the JavaScript source code in the file index.html. Notice the placeholder for the back-end API server (##QOTD_API_URL##):
function loadURL() { var url = localStorage.getItem("apiURL"); if (!url) { url = '##QOTD_API_URL##'; localStorage.setItem("apiURL", url); }; document.getElementById("urlLink").innerText = url; };
- The solution is to use a Bash shell command, sed, to change the contents of this file before launching the Nginx server. In conjunction with this, the Dockerfile (or Containerfile) used to build the image, employs the CMD function to call a Bash script. Here’s an example of the Dockerfile:
FROM docker.io/nginx WORKDIR /usr/share/nginx/html COPY nginx.conf /etc/nginx/nginx.conf COPY *.* . RUN chmod +x ./container_run.sh RUN chmod 777 ./ RUN chown -R 1001:0 /var/cache/nginx && chmod -R ug+rwx /var/cache/nginx && chown -R 1001:0 /var/run RUN chown -R 1001:0 /run USER 1001 EXPOSE 8080 CMD ./container_run.sh The file “container_run.sh” has the following contents: # This script will replace the value in the file index.html. # By replacing the value with the contents of the environment variable, # we are able to specify the qotd webapi URL at runtime. # E.g. podman run -e QOTD_API_URL="https://foo/bar/quotes/random" quay.io/donschenck/qotd-frontend:latest sed -i "s|##QOTD_API_URL##|$QOTD_API_URL|g" index.html nginx -g "daemon off;"
Congratulations. You're done with this lesson. Now it's time to combine your image-build with this script, so you can change the source code before running the server.