Page
Ansible Playbook variables and facts
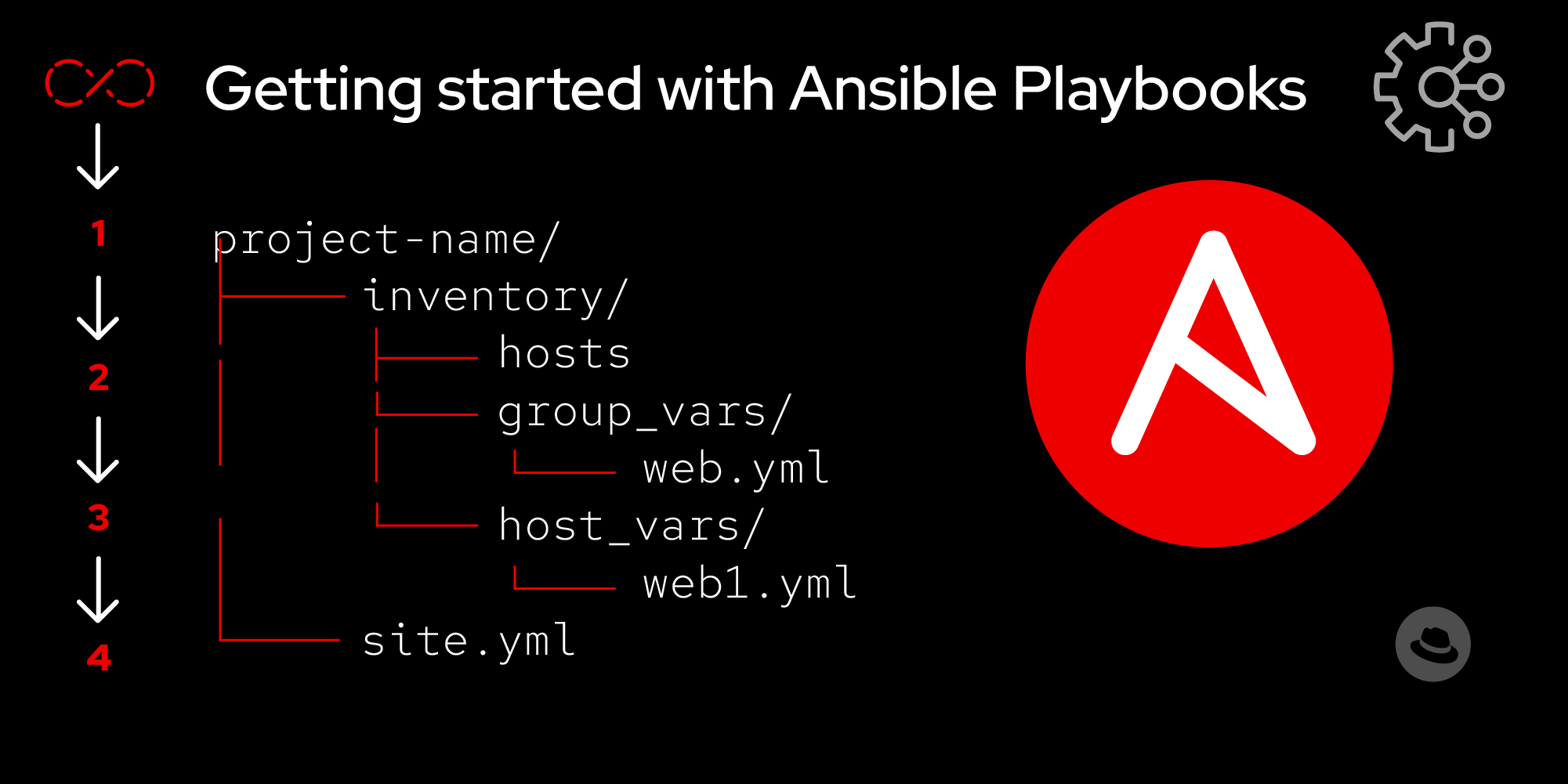
Now that you have learned more about Ansible’s directory structure and inventories let us have a look at the variables in Ansible and how Ansible facts can be used to retrieve detailed information of managed hosts.
In this lesson, you will:
- Learn about variables in Ansible.
- Learn about Ansible facts.
Variables in Ansible
Variables in Ansible are YAML key-value pairs that store data for reuse in playbooks, making them more flexible and customizable. You can define variables in your playbooks, inventory, variable files, or at the command line.
Review the official Ansible documentation for information on variable precedence.
Declaring variables in playbooks
Variables in playbooks are defined under the vars section in a play and can be used in the specific play they’re declared in.
Let’s add variables to our site.yml
playbook:
---
- name: Install and start Apache
hosts: web
become: true
vars:
package_name: httpd
tasks:
- name: Ensure the httpd package is installed
ansible.builtin.package:
name: "{{ package_name }}"
state: present
Ansible playbook variable example.
Code | Definition |
| The |
| Instead of using |
Using variables in the Inventory
The previous lesson covered adding variables to Ansible inventory groups and hosts. Let’s use an example to illustrate using these variables in a playbook.
---
all:
children:
web:
vars:
package_name: httpd
hosts:
web1:
ansible_host: 192.168.0.10
web2:
ansible_host: web2.example.com
database:
hosts:
db1:
ansible_user: rhel
Ansible YAML inventory variables example.
Code | Definition |
| There is a new vars section in the web inventory group. |
| We’ve created the package_name variable and set its value to |
We can reuse the package_name
variable in our playbook:
---
- name: Install and start Apache
hosts: web
become: true
tasks:
- name: Ensure the httpd package is installed
ansible.builtin.package:
name: "{{ package_name }}"
state: present
Ansible playbook inventory variables example.
Code | Definition |
| This playbook will install the |
Using variable files
Variable files are key-value pairs commonly created in YAML files. Using variable files could make your playbooks easier to reuse and maintain.
# vars.yml
---
package_name: httpd
Variable file example.
You can include variable files in your playbook using the vars_files
section or the ansible.builtin.include_vars module.
Let’s use an example to include a variable file in a playbook:
---
- name: Install and start Apache
hosts: web
become: true
vars_files:
- vars.yml
tasks:
- name: Ensure the httpd package is installed
ansible.builtin.package:
name: "{{ package_name }}"
state: present
Ansible playbook variable file example.
Code | Definition |
| The vars_files section includes the |
| The |
Using host_vars and group_vars
Ansible automatically loads variable files into playbooks in the host_vars
and group_vars
folders based on the host or group name and the associated file name.
For Ansible to automatically associate the host_vars
and group_vars
folders, they must be in a specific location relative to your Ansible inventory file or playbook file.
The folders can be adjacent to the inventory file:
project-name/
├── inventory/
│ ├── hosts # Custom inventory file
│ ├── group_vars/
│ │ └── web.yml # Variables for 'web group'
│ └── host_vars/
│ └── web1.yml # Variables for 'web1 host'
└── site.yml
Ansible inventory folder group_vars and host_vars.
Or the folders can be adjacent to the Ansible playbook:
project-name/
├── inventory # Inventory file
├── group_vars/
│ └── web.yml # Variables for 'web' group
├── host_vars/
│ └── web1.yml # Variables for 'web1' host
└── site.yml
Ansible playbook adjacent folder group_vars and host_vars.
For example, let’s create a web.yml
YAML file in the ./project-name/group_vars/
folder:
# ./project-name/group_vars/web.yml
---
package_name: httpd
Ansible inventory group_vars example.
Code | Definition |
| The |
Ansible facts
You can retrieve detailed information about managed nodes or about Ansible itself. These data points are called Ansible facts. Facts let you adapt the playbook to the specific characteristics of managed nodes without complex, hard-coded logic.
Unless the gather_facts
option is disabled, Ansible gathers facts at the beginning of each play execution and contains information about a host’s filesystem, network interfaces, and more.
Facts are stored in the ansible_facts
variable and can be accessed in playbooks.
---
- name: Install and start Apache
hosts: web
become: true
vars_files:
- vars.yml
tasks:
- name: Filter and return only selected facts
ansible.builtin.setup:
filter:
- 'os_family'
- 'ansible_distribution'
- name: Ensure the httpd package is installed
ansible.builtin.package:
name: "{{ package_name }}"
state: present
when: ansible_facts['os_family'] == "RedHat"
Ansible playbook facts example.
Code | Definition |
| You can gather facts by using the ansible.builtin.setup module or running |
| We are using a conditional statement to install the |
Next, we’ll cover more advanced Ansible playbook topics: handlers, conditionals, and Jinja2 templates.