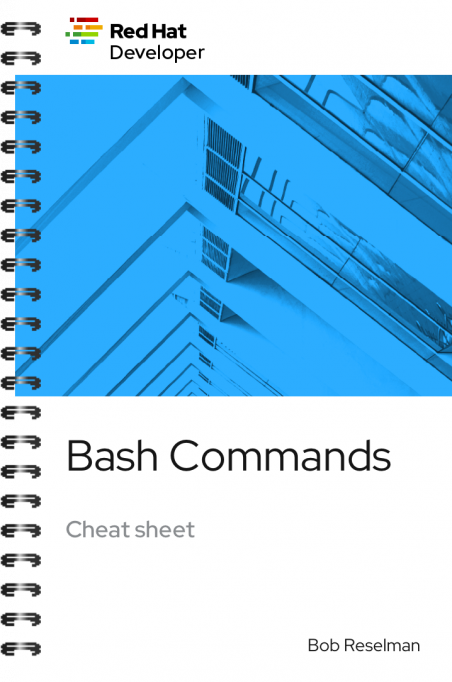
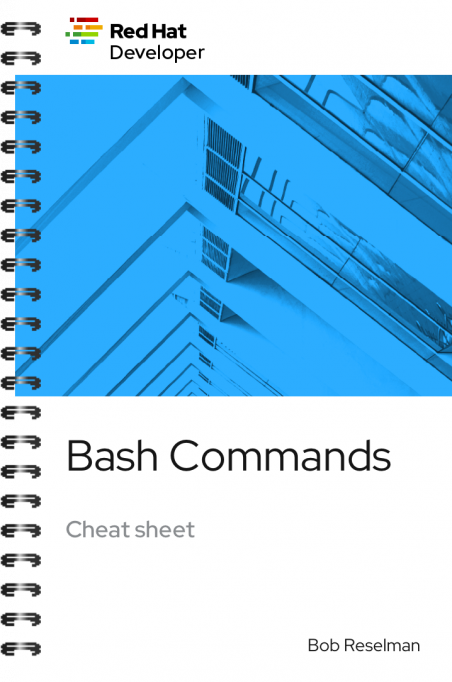
About
Get an easy-to-understand introduction to Bash, the powerful command-line shell and scripting language for Linux. With Bash scripting, you can automate repetitive tasks, manage system operations, and boost your overall coding productivity.
Our Bash scripting cheat sheet is a quick guide to running Bash scripts, explaining foundational concepts through helpful code examples. You'll learn how to perform the following tasks:
- Create Bash scripts using shebang headers or by directly calling the Bash interpreter
- Declare variables
- Manipulate strings using parameter expansion
- Manage arrays and maps
- Define functions for reusing code
- Create conditional statements for decision-making
- Use loops to process data
- Report success and failure using exit codes
Download the Bash cheat sheet and learn the basics of Bash shell scripting to start automating tasks efficiently within your Linux environment.
Red Hat Developer cheat sheets give you essential information right at your fingertips so you can work faster and smarter. Easily learn new technologies and coding concepts and quickly find the answers you need.
Excerpt
Creating an array
The following creates an array with three elements and assigns the array to the variable named my_array
.
my_array=('Alex' 'Ada' 'Alexandra')
Adding an element to an array
The following uses the +=
operator to add an element with the value Soto
to the array named my_array
.
my_array+=('Soto')
Removing an element to an array
The following uses the unset
keyword to remove the fourth element from the array named my_array
at index 3
.
unset my_array 3