This article is the first in a two-part series on Kubernetes configuration patterns, which represent ways of configuring Kubernetes applications and controllers. Part 1 introduces simple approaches that use only Kubernetes primitives. These patterns are applicable to any application running on Kubernetes. Part 2 introduces more advanced patterns. These patterns require you to code against the Kubernetes API when you are developing Kubernetes controllers.
Note: Kubernetes controllers are programs that run on Kubernetes and manage resources in Kubernetes.
For simplicity, I've used only Deployment
s in the example YAML files. However, the examples should work with other PodSpecable
s (anything that describes a PodSpec
) such as DaemonSet
s and ReplicaSet
s. I also omitted fields like image
, imagePullPolicy
, and others in the example Deployment
YAML.
Configuration with command-line arguments
Kubernetes allows you to pass command-line arguments to containers, which can be facilitated to provide configuration to the applications:
apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: containers: - name: server args: - "gravity=10" - "colorMode=dark"
The command-line arguments gravity
and colorMode
will be passed to the application running in the container. The application program needs to read the command-line arguments and apply them.
This Kubernetes configuration pattern is not very flexible: Configuration is hardcoded in the YAML, so the configuration is coupled to the Deployment
. Also, this pattern doesn’t scale well when the same configuration is needed in multiple Deployment
s.
This practice can be used for migrating legacy software that uses command-line arguments for configuration into containers. However, any of the alternatives presented in this article would be better in terms of flexibility and scalability.
Configuration with environment variables
Kubernetes allows you to set environment variables in containers. Applications in containers may use these environment variables as configuration options. For example:
apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: template: spec: containers: - name: server env: - name: GRAVITY value: "10" - name: COLOR_MODE value: "dark"
The environment variables GRAVITY
and COLOR_MODE
will be passed to the application running in the container. The application program needs to read the environment variables and apply them. The Deployment
will be rolled out when there is a change in the environment variables.
This pattern is almost identical to the previous one, Configuration with command-line arguments, as the configuration is still hardcoded in the Deployment
YAML. However, environment variables are easier and cleaner to define and provide to containers than command-line arguments. Also, in the command-line arguments approach, the configuration and the command to run the application are in the same place.
As you will see soon, Kubernetes also lets you pass environment variables to get values from different sources, such as Secret
s, ConfigMap
s, and references.
If you don't need to share the configuration among different Deployment
s or containers, this pattern provides the simplest and most straightforward configuration method.
Antipattern: Configuration with NFS volume mounts
It is possible to mount network drives to containers with Kubernetes.
In this pattern, the idea is to mount a volume with a configuration file in it to a container. Later, the application running in the container can read the configuration file from the file system.
To create such volume, we can use a network file system (NFS) volume, as shown in Figure 1.
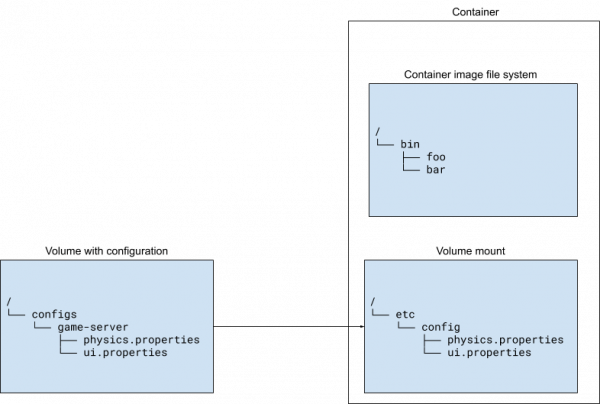
Here is the part of the Deployment
for implementing the mount:
apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: volumes: - name: nfs-volume nfs: server: nfs.example.com path: /configs/game-server/ containers: - name: server volumeMounts: - name: nfs-volume mountPath: /etc/config
In this example, the game-server
container will be able to read the files under the /etc/config/
directory, and that directory will be a reflection of the /configs/game-server/
path in the NFS server that is defined in the Deployment
.
You need to create the configuration files manually on the NFS server before creating the Deployment
on Kubernetes.
This pattern is very similar to the Configuration with a ConfigMap mounted as a file pattern, which I will show shortly. The difference here is that you need to use NFS and create the configuration file manually on the NFS server. Because of these requirements, this solution is an antipattern, although in some cases it is useful. One of the cases is when the configuration is very large, such as machine learning models (if they can be considered part of configuration). Another useful case is when the configuration must be immutable.
Antipattern: Configuration with init containers
Kubernetes has a concept of init containers. These containers run before the main containers and configure the environment for them. You can exploit this feature to handle an application's configuration.
In this pattern, the configuration lives in a separate image that is run by an init container. That container copies the configuration it holds into a volume shared with other containers, as illustrated by Figure 2. The init container holds the configuration and later copies the configuration over to the volume that is shared with the server container.
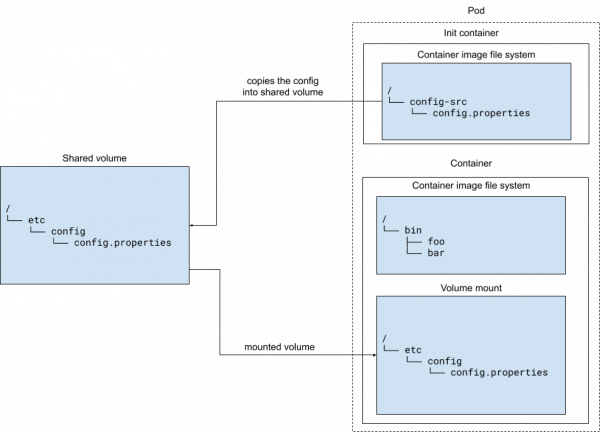
Here is the part of the Deployment
for implementing the init container:
apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: volumes: - name: config-directory emptyDir: {} initContainers: - name: init image: my.image-repository.com/initcontainer volumeMounts: - name: config-directory mountPath: /etc/config containers: - name: server image: my.image-repository.com/maincontainer volumeMounts: - name: config-directory mountPath: /etc/config
You need to build the init container that way, though. For example:
FROM busybox ADD configuration.properties /config-src/configuration.properties ENTRYPOINT [ "sh", "-c", "cp /config-src/* /etc/config", "--" ]
The config.properties
file is the configuration file that is added to the container image during its build. Later, the file is simply copied with the cp
command. So, the configuration should be available during the init container's build time.
Any changes done to the configuration file by the server container in the shared volume will be transient. Also, having the configuration available during the build time is not very flexible and not 12-factor-ish.
You can use this pattern in situations similar to those for the Configuration with NFS volume mounts pattern, when the configuration file is too large or if an immutable configuration is targeted. But using an init container as shown here is considered an antipattern, except for these specific cases.
Configuration with a ConfigMap mounted as a file
It is possible to mount a Kubernetes ConfigMap
into a container as a file, as shown in Figure 3.
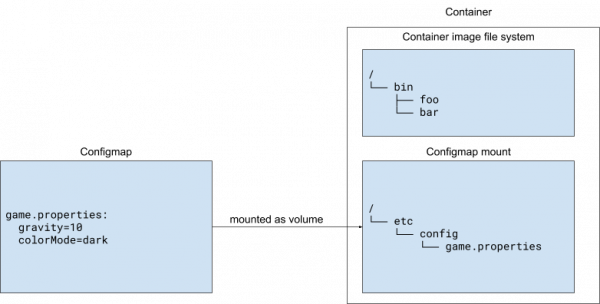
Here is the part of the Deployment
for mounting the ConfigMap
:
apiVersion: v1 kind: ConfigMap metadata: name: game-config data: game.properties: | gravity=10 colorMode=dark --- apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: containers: - name: server volumeMounts: - mountPath: /etc/config name: game-config-volume volumes: - name: game-config-volume configMap: name: game-config
The data inside the ConfigMap
will be mounted to the Deployment
as a file in a volume. The key in the data field, game.properties
, is used as the file name, and the value of that key will be the file content. The configuration file path will be /etc/config/game.properties
. When you follow this procedure, the game application can read and process the .properties
file.
This configuration pattern is easy to understand and manage. The configuration can be read as a whole file in the application container, which might be simpler and faster. Also, the same ConfigMap
can be mounted to different containers easily, compared to setting values from ConfigMap
s as environment variables.
With this pattern, the configuration is loosely coupled from the application. The configuration can be changed without touching the Deployment
. It is possible to use the same configuration in multiple applications as well as to mount multiple ConfigMap
s into a single Deployment
. Multiple ConfigMap
s provide more granularity when needed.
However, this pattern needs a file read operation within the application running in the container, which might not be ideal in every case. Furthermore, when using this pattern, the configuration that the Deployment
is using is not visible directly on the Deployment
or the Pod
s created for the Deployment
. A human operator needs to check the ConfigMap
to see the configuration values.
When there are a lot of configuration options, a ConfigMap
mount makes sense because the configuration is available as a whole with a single volumeMount
. Also, when a specific file format is used, such as the .properties
file in the previous example, the file content can be fed to a properties file parser, which is available in many languages.
By default, the Deployment
will not be restarted when there is a change in the mounted ConfigMap
. There are techniques to restart the Deployment
, but they are outside the scope of this article.
When there are not many configuration options, you can use alternative patterns like Setting environment variables from ConfigMaps (shown below) to avoid the file read operation and the volume mount.
You can make a mounted ConfigMap
read only, but not immutable. Cluster administrators will be able to change the ConfigMap
, and thus the configuration for an application. If immutability is needed, use the Configuration with NFS volume mounts or Configuration with init containers pattern.
Configuration with ConfigMaps mounted as multiple files
When a ConfigMap
contains multiple keys, it is possible to mount these keys as separate files.
This pattern is the same as the Configuration with a ConfigMap mounted as a file pattern, except that it uses multiple files to separate parts of the configuration, thus creating different scopes. Figure 4 shows this pattern.
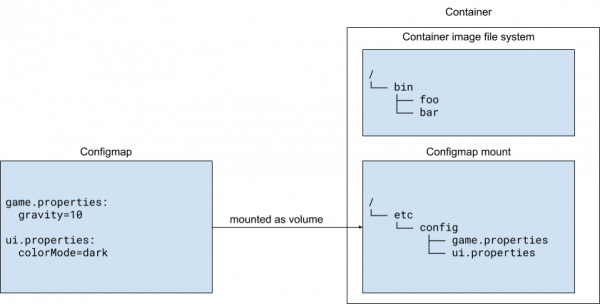
Here is the part of the Deployment
for creating the ConfigMap
s and volume:
apiVersion: v1 kind: ConfigMap metadata: name: game-config data: game.properties: | gravity=10 ui.properties: | colorMode=dark --- apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: containers: - name: server volumeMounts: - mountPath: /etc/config name: game-config-volume volumes: - name: game-config-volume configMap: name: game-config
As in the Configuration with a ConfigMap mounted as a file pattern, the data inside the ConfigMap
will be mounted to the Deployment
as files in a volume. This time, though, there will be two files: /etc/config/game.properties
and /etc/config/ui.properties
.
This separation is useful in some cases, such as when application modules read their configurations independently, or when the configuration has so many options that it makes sense to split the options into multiple logical parts while keeping everything in a single ConfigMap
.
Configuration with ConfigMaps used as a source for environment variables
This pattern is preferred when there are not many configuration options and when I/O operations are undesired. Figure 5 shows the pattern.
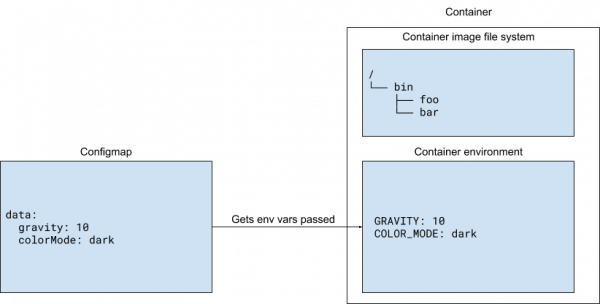
Here is the part of the Deployment
for implementing the ConfigMap
and environment variables:
apiVersion: v1 kind: ConfigMap metadata: name: game-config data: gravity: "10" colorMode: "dark" --- apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: containers: - name: server env: - name: GRAVITY valueFrom: configMapKeyRef: name: game-config key: gravity - name: COLOR_MODE valueFrom: configMapKeyRef: name: game-config key: colorMode
The environment variables will be set with the values from ConfigMap
, ending up as GRAVITY=10
and COLOR_MODE=dark
. The application running in the container needs to read the environment variables and use them as the configuration.
This pattern provides a nice way to bind the options in the ConfigMap
to the configuration options that are actually used in the application. There might be more options in the ConfigMap
, but not every application needs all of the options.
The mappings from ConfigMap
keys to environment variables are explicit and make these bindings human readable. Furthermore, the application does not need any file I/O operations.
However, if there are too many configuration options that need to be bound from a ConfigMap
to environment variables, things can get messy. In that case, the Configuration with a ConfigMap mounted as a file pattern is preferred.
Configuration with Secrets
Anything done for configuration can theoretically be done with Secret
s. It is better to store credentials, certificates, and similar things in a Secret
than in a ConfigMap
, because Secret
s are more secure.
For example, to mount a Secret
as a file on a volume, you can use the following YAML:
apiVersion: v1 kind: Secret type: Opaque metadata: name: game-secret data: databaseUsername: Zm9v databasePassword: YmFy --- apiVersion: apps/v1 kind: Deployment metadata: name: game-server-deployment spec: ... template: spec: containers: - name: server volumeMounts: - mountPath: /etc/config name: game-secret-volume volumes: - name: game-secret-volume configMap: name: game-secret
This will create two files that are available to the server container: /etc/config/databaseUsername
and /etc/config/databasePassword
.
As shown in this example, simply replacing the ConfigMap
with Secret
should work most of the time.
Conclusion to Part 1
The Kubernetes configuration patterns described in this article use Kubernetes primitives and will help you configure your application running on Kubernetes. They are relatively simple to implement. The second part of this article introduces patterns specific to Kubernetes controllers. These will require coding against the Kubernetes API.
Last updated: October 14, 2022