Spring and Spring Boot are developer favorites for building Java applications that run in the cloud. Spring is known for being production-ready, complimenting containerization very well. According to the State of Spring 2022 report, Kubernetes has become the dominant platform for running Spring apps. So, how can we take a Spring application, containerize it, and run it locally? Let’s explore this by using Podman Desktop, an open-source tool to seamlessly work with containers and Kubernetes from your local environment.
Prerequisites
- Spring Boot Application: For this article, we’ll use the popular Spring PetClinic sample application on GitHub (Figure 1). Feel free to also use your own project or start from the Spring Initializr.
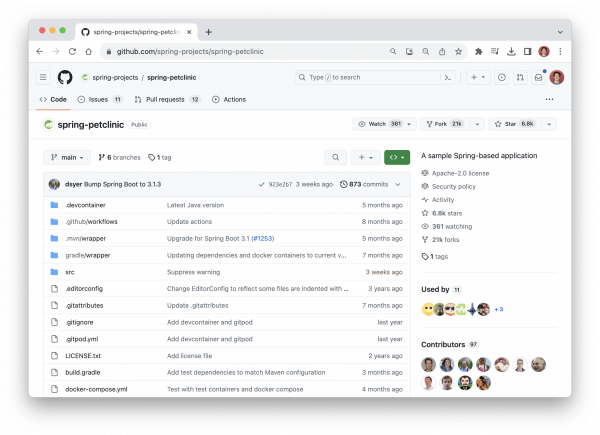
- Podman Desktop: Let’s use Podman Desktop, the powerful GUI-based tool for deploying and managing containers using the Podman container engine. Once installed, you’ll be ready to start containerizing your Spring application (Figure 2).
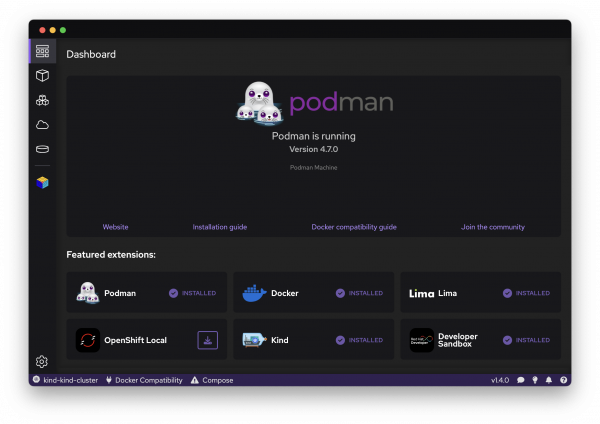
Containerizing the Spring Boot application
Let’s get started by cloning the application's source code if using the PetClinic repository.
git clone https://github.com/spring-projects/spring-petclinic.git cd spring-petclinic
While we can use Maven to build a jar file and run it, let’s jump straight into creating a Containerfile in the project’s root directory, which will serve as the blueprint for the container image we’ll create later (analogous to Docker’s Dockerfile). You can create the file with the command touch Containerfile
or simply create it from your IDE. Let’s see what the Containerfile will look like for this sample Spring Boot application:
# Start with a base image that has Java 17 installed. FROM eclipse-temurin:17-jdk-jammy # Set a default directory inside the container to work from. WORKDIR /app # Copy the special Maven files that help us download dependencies. COPY.mvn/.mvn # Copy only essential Maven files required to download dependencies. COPY mvnw pom.xml./ # Download all the required project dependencies. RUN./mvnw dependency:resolve # Copy our actual project files (code, resources, etc.) into the container. COPY src./src # When the container starts, run the Spring Boot app using Maven. CMD ["./mvnw", "spring-boot:run"]
Let’s take a deeper look at the components that make up this Containerfile:
-
FROM eclipse-temurin:17-jdk-jammy:
- Purpose: This line sets the foundation for our container.
- Deep Dive: It tells Podman to use a pre-existing image that already has Java 17 installed. Think of it as choosing a base flavor for a cake before adding more ingredients. We use the eclipse-temurin image because it’s a trusted source for Java installations.
-
WORKDIR /app:
- Purpose: Designates a working space in our container.
- Deep Dive: Containers have their own file system. Here, we're telling Podman to set up a folder named 'app' and make it the default location for any subsequent operations.
-
COPY commands:
- Purpose: They transfer files from our local system into the container.
- Deep Dive: The first COPY grabs Maven’s configuration, a tool Java developers use to manage app dependencies. The second copies over the main files of our app: the Maven wrapper (mvnw) and the project's details (pom.xml).
-
RUN./mvnw dependency:resolve:
- Purpose: Downloads necessary libraries and tools for the app.
- Deep Dive: This command activates Maven to fetch everything our app needs to run. By doing this in the Containerfile, we ensure the container has everything packaged neatly.
-
COPY src./src:
- Purpose: Add our actual application code.
- Deep Dive: Our app's logic, features, and resources reside in the src directory. We're moving it into the container so that when the container runs, it has our complete app.
-
CMD ["./mvnw", "spring-boot:run"]:
- Purpose: Start our application!
- Deep Dive: This command is the final step. This line tells Podman to run our Spring Boot application using Maven when our container launches.
This Containerfile is ready to be used with Podman Desktop to create a container image of our Spring Boot application (Figure 3). Before building the image for this application, let's double check the directory structure and Containerfile in our IDE of choice.
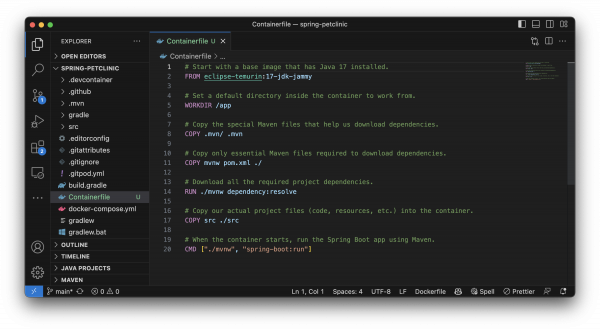
Building the container image with Podman Desktop
We can now build our container image with Podman Desktop by first heading to the Images section of Podman Desktop and selecting the Build an Image button in the top-right corner (Figure 4).
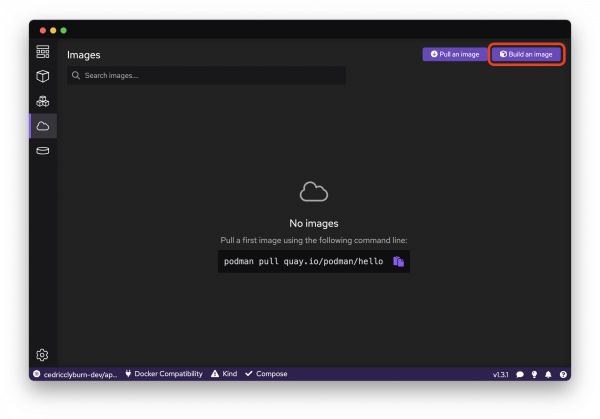
This will open a menu where you can select the path to our previously created Containerfile, which should be in the root directory of the spring-petclinic folder. With the Containerfile selected, we can also give our container image a name, for example, petclinic. Now, click on Build, and you’ll see each of the layers of the image being created. You can find this in your local image registry (the Image section in Figure 5).
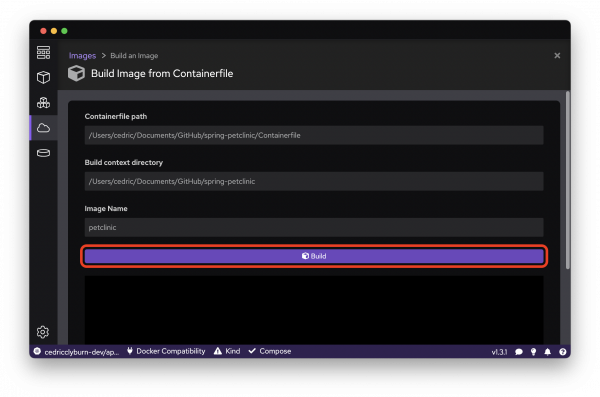
Running the containerized application
Fantastic! Let’s return to the Images section to see the containerized Spring Boot application, now built and tagged as an image, as well as the eclipse-temurin base image that was downloaded to build our petclinic image. We can easily run this image as a container on our system using the Run icon to the right of our container image (Figure 6).
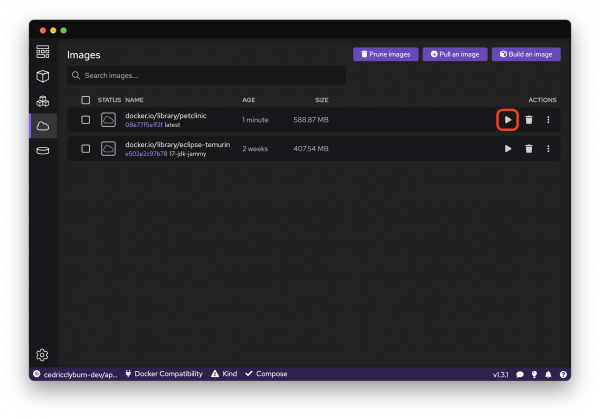
Under Port Mapping, make sure to assign port 8080 of the container to port 8080 of the host. Feel free to leave all other settings as default. Click Start Container to launch the containerized instance of your Spring Boot application (Figure 7).
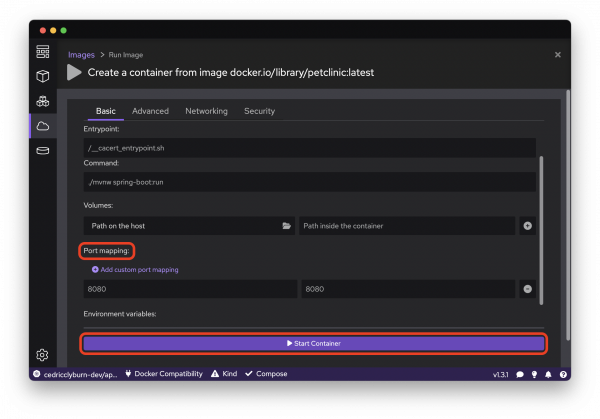
Now, with the container up and running, let’s open the container’s URL in the browser using the handy open browser button within the Podman Desktop user interface (Figure 8).
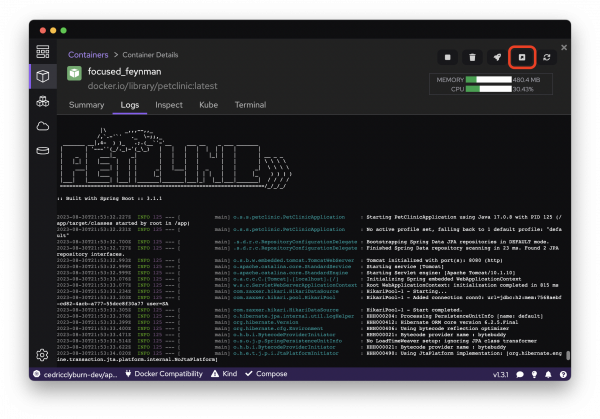
Perfect, looks like our Spring Boot application is running, thanks to the startup command in our Containerfile, as well as the port mapping we configured in Podman Desktop (Figure 9).
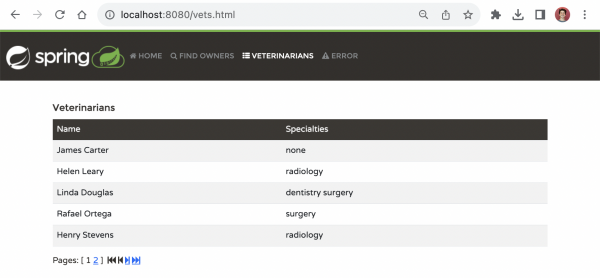
Now we can see this PetClinic application running in our browser, but that’s not all. For more features that may involve bringing out the terminal, we can now use Podman Desktop instead (Figure 10). This may include, SSH’ing into a container for debugging and modifying settings, maybe viewing the logs of a container, or inspecting environment variables. This can all be done under the Container Details section that opens automatically after starting the container.
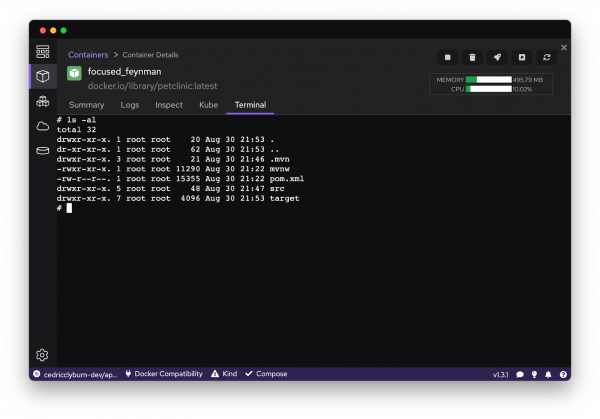
Wrapping up
We have gone from local Spring Boot code to containerizing the application and running it with Podman Desktop! Now, as a container, we can share this application across environments using registries like Quay.io and Docker Hub and deploy it using Kubernetes and OpenShift to a variety of different cloud providers.
Last updated: October 25, 2023